- Published on
Unit testing in Go
2 min read
- Authors
- Name
- Karan Pratap Singh
- @karan_6864
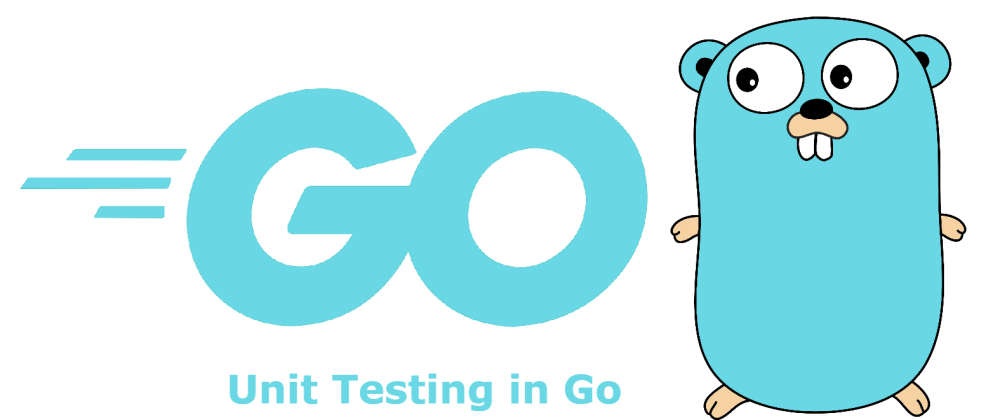
Table of Contents
In this article let's discuss how we can write simple unit tests in go.
All the code from this article is available on github
I've also made a video, if you like to follow along!
Writing our first unit test
Initialize a sample
module
go mod init sample
Let's start with adding a length.go
file with a Length
function. This function simply returns the length of the input string.
In go, tests file are usually named with _test
(just like we do .test.js
for jest). Let's create length_test.go
.
This is a very basic test function in which we'll be testing out our Length
function
Let's add some test logic
Let's run it!
go test
Yay! our test passed!
PASS
ok sample 0.429s
Now let's change our expected
variable to 5
, and re-run our test and we should see the test fail
--- FAIL: Test (0.00s)
length_test.go:10: Expected 5 Got 4
FAIL
exit status 1
FAIL sample 0.334s
Multiple test cases
Let's add out test cases with input
and expected
outputs
This approach is similar to test.each
from jest
Update Test_Length
function to use testCases
Run the test cases!
PASS
ok sample 0.347s
Great! Thank you all for reading this article, if you have any questions don't hesitate to reach to me on twitter